728x90
반응형
로블록스(Roblox) 게임을 만들려면 Roblox Studio를 사용해야 합니다. 기본적인 과정은 다음과 같습니다.
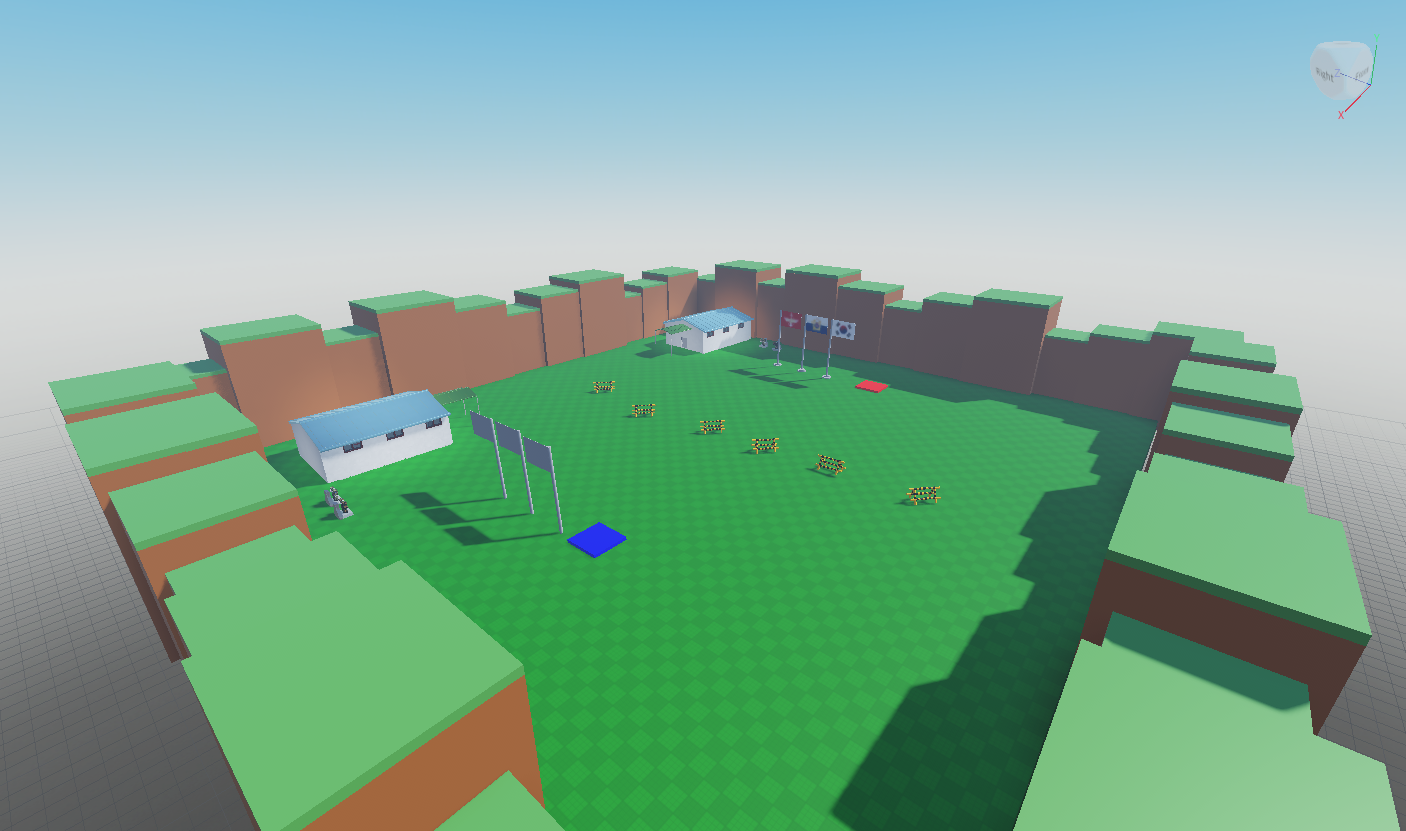
1. 로블록스 스튜디오 설치 및 실행
- 로블록스 공식 웹사이트에서 Roblox Studio 다운로드.
- 설치 후 로그인하여 실행.
2. 새 게임 프로젝트 생성
- Roblox Studio를 열고 "New"(새 프로젝트) 선택.
- 기본 템플릿 중 Baseplate(기본 판) 또는 Obby(장애물 코스) 선택.
3. 게임 환경 만들기
① 지형 및 오브젝트 추가
- Toolbox(도구 상자)에서 블록, 건물, 아이템 추가.
- Part 도구를 사용하여 직접 오브젝트 제작 가능.
- Terrain Editor를 사용해 산, 물, 땅 등의 지형 추가 가능.
② 캐릭터와 이동 시스템 추가
- 기본적으로 플레이어가 걷고 점프할 수 있음.
- StarterPlayer 속성에서 캐릭터 이동 속도, 점프력 조절 가능.
4. 게임 스크립트 작성 (Lua)
① 기본 스크립트 추가 방법
- Explorer(탐색기) 창에서 ServerScriptService 또는 StarterPlayerScripts 선택.
- Script 추가 → Lua 코드 작성.
② 예제: 점프하면 "점프했다!" 출력
local player = game.Players.LocalPlayer
player.CharacterAdded:Connect(function(character)
local humanoid = character:WaitForChild("Humanoid")
humanoid.Jumping:Connect(function()
print("점프했다!")
end)
end)
player.CharacterAdded:Connect(function(character)
local humanoid = character:WaitForChild("Humanoid")
humanoid.Jumping:Connect(function()
print("점프했다!")
end)
end)
5. 게임 테스트 및 수정
- 상단 "Play" 버튼 클릭하여 직접 플레이하며 오류 확인.
- 오류 발생 시 Output 창에서 디버깅.
6. 게임 퍼블리싱 (출시)
- File → Publish to Roblox 선택.
- 게임 이름, 설명 입력 후 업로드.
- 설정에서 Public(공개) 으로 변경하면 다른 플레이어들도 플레이 가능.
🚀 1. 체크포인트 시스템 (Obby 게임용)
🔹 플레이어가 체크포인트를 지나면 저장되는 기능입니다.
🔹 StarterPlayerScripts에 아래 코드를 추가하세요.
local checkpoints = game.Workspace:FindFirstChild("Checkpoints") -- Checkpoints 폴더 가져오기
local players = game:GetService("Players")
local function onTouched(part)
local character = part.Parent
local player = players:GetPlayerFromCharacter(character)
if player then
local checkpoint = part.Parent
player:SetAttribute("Checkpoint", checkpoint.Position) -- 체크포인트 저장
print(player.Name .. "가 체크포인트에 도착했습니다!")
end
end
for _, checkpoint in pairs(checkpoints:GetChildren()) do
if checkpoint:IsA("Part") then
checkpoint.Touched:Connect(onTouched)
end
end
local players = game:GetService("Players")
local function onTouched(part)
local character = part.Parent
local player = players:GetPlayerFromCharacter(character)
if player then
local checkpoint = part.Parent
player:SetAttribute("Checkpoint", checkpoint.Position) -- 체크포인트 저장
print(player.Name .. "가 체크포인트에 도착했습니다!")
end
end
for _, checkpoint in pairs(checkpoints:GetChildren()) do
if checkpoint:IsA("Part") then
checkpoint.Touched:Connect(onTouched)
end
end
✅ 적용 방법:
- Explorer 창에서 Checkpoints 폴더를 만들고, 그 안에 Part(블록) 추가.
- 위 스크립트 적용하면, 플레이어가 체크포인트를 밟으면 저장됨.
🔫 2. 간단한 무기 시스템 (PVP용)
🔹 Tool(무기)를 추가하고, 플레이어가 클릭하면 총알이 발사되도록 설정
🔹 StarterPack에 아래 스크립트를 추가하세요.
local tool = script.Parent
tool.Activated:Connect(function()
local character = tool.Parent
local humanoid = character:FindFirstChild("Humanoid")
if humanoid then
print("총을 발사했습니다!")
-- 총알 만들기
local bullet = Instance.new("Part")
bullet.Size = Vector3.new(0.5, 0.5, 2)
bullet.Color = Color3.new(1, 0, 0)
bullet.Material = Enuhttp://m.Material.Neon
bullet.CFrame = character.Head.CFrame + character.Head.CFrame.LookVector * 2
bullet.Parent = game.Workspace
-- 총알 이동
local velocity = Instance.new("BodyVelocity")
velocity.Velocity = character.Head.CFrame.LookVector * 50
velocity.Parent = bullet
-- 3초 후 총알 삭제
game:GetService("Debris"):AddItem(bullet, 3)
end
end)
tool.Activated:Connect(function()
local character = tool.Parent
local humanoid = character:FindFirstChild("Humanoid")
if humanoid then
print("총을 발사했습니다!")
-- 총알 만들기
local bullet = Instance.new("Part")
bullet.Size = Vector3.new(0.5, 0.5, 2)
bullet.Color = Color3.new(1, 0, 0)
bullet.Material = Enuhttp://m.Material.Neon
bullet.CFrame = character.Head.CFrame + character.Head.CFrame.LookVector * 2
bullet.Parent = game.Workspace
-- 총알 이동
local velocity = Instance.new("BodyVelocity")
velocity.Velocity = character.Head.CFrame.LookVector * 50
velocity.Parent = bullet
-- 3초 후 총알 삭제
game:GetService("Debris"):AddItem(bullet, 3)
end
end)
✅ 적용 방법:
- Explorer에서 StarterPack 폴더를 찾기
- Tool 객체를 추가하고, 안에 Script 추가
- 위 코드 적용 후 플레이어가 무기를 들고 클릭하면 총알 발사됨!
🧟 3. 좀비 NPC AI (적 공격하기)
🔹 좀비가 플레이어를 따라오고, 근접하면 공격하는 기능 추가.
🔹 ServerScriptService에 아래 스크립트를 추가하세요.
local zombie = script.Parent
local humanoid = zombie:FindFirstChild("Humanoid")
local players = game:GetService("Players")
local target = nil
-- 플레이어 찾기
local function findTarget()
local nearestDistance = math.huge
for _, player in pairs(players:GetPlayers()) do
if player.Character then
local distance = (player.Character.PrimaryPart.Position - zombie.PrimaryPart.Position).magnitude
if distance < nearestDistance then
nearestDistance = distance
target = player.Character
end
end
end
end
-- 움직이기
while true do
findTarget()
if target then
humanoid:MoveTo(target.PrimaryPart.Position)
end
wait(1)
end
local humanoid = zombie:FindFirstChild("Humanoid")
local players = game:GetService("Players")
local target = nil
-- 플레이어 찾기
local function findTarget()
local nearestDistance = math.huge
for _, player in pairs(players:GetPlayers()) do
if player.Character then
local distance = (player.Character.PrimaryPart.Position - zombie.PrimaryPart.Position).magnitude
if distance < nearestDistance then
nearestDistance = distance
target = player.Character
end
end
end
end
-- 움직이기
while true do
findTarget()
if target then
humanoid:MoveTo(target.PrimaryPart.Position)
end
wait(1)
end
✅ 적용 방법:
- Explorer에서 Zombie 모델 만들기
- Humanoid 추가 (움직이도록 설정)
- 위 코드 적용 후 실행하면 좀비가 플레이어를 따라감!
728x90